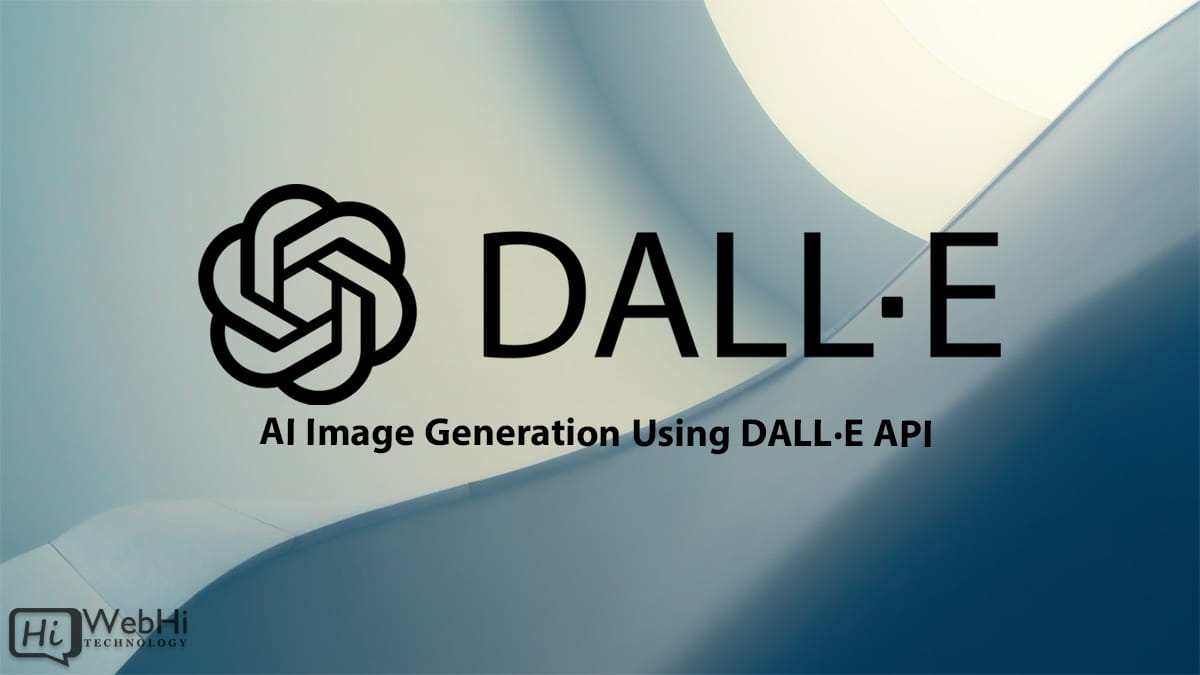
Artificial Intelligence (AI) has transformed a wide array of industries, and one of the most exciting applications is in creative fields such as image generation. OpenAI’s DALL·E API brings this to the forefront, allowing developers and artists to create unique and high-quality images based on text prompts. The DALL·E model, specifically its latest iteration (DALL·E 3), has taken the world by storm due to its ability to understand complex and nuanced descriptions and generate realistic and imaginative images.
This comprehensive guide will help you get started with the DALL·E API and show you how to integrate AI-generated image functionality into your applications, making it easy to generate custom images directly from textual descriptions.
Table of contents
- Introduction to DALL·E API
- Setting Up Your Environment
- Understanding the DALL·E API
- Generating Images with DALL·E
- Advanced Features and Capabilities
- Best Practices for Effective Image Generation
- Integrating DALL·E into Your Applications
- Troubleshooting Common Issues
- Conclusion
1. Introduction to DALL·E API
DALL·E is an AI model developed by OpenAI that is capable of generating images from natural language descriptions. The model has evolved from its first version to DALL·E 2, and now, DALL·E 3, which brings even more power and sophistication in terms of handling complex prompts, generating high-quality visuals, and interpreting nuances in text.
What is DALL·E?
DALL·E is a neural network trained to generate images from text descriptions. This allows users to create images of objects, environments, or abstract concepts that may not exist in the real world, all based on a simple text prompt. For example, you can input a phrase like “a purple elephant riding a skateboard,” and DALL·E will generate an image of exactly that. This technology has huge potential for industries like gaming, marketing, e-commerce, and even content creation.
What is the DALL·E API?
The DALL·E API allows developers to integrate the power of DALL·E into their applications. By using the API, you can generate images based on textual input programmatically. OpenAI has provided this tool for developers, artists, and researchers to experiment with AI-driven image generation in various creative and business applications.
2. Setting Up Your Environment
Before you begin generating images with the DALL·E API, it’s important to set up your development environment correctly. Below, we cover the steps to ensure that you have everything needed to get started.
2.1. Prerequisites
- Python 3.7 or higher: Ensure that Python is installed on your system. You can verify this by running:
$ python --version
- OpenAI Account: You will need an OpenAI account to access the API. If you don’t already have one, sign up at OpenAI’s website.
- API Key: After signing up, you’ll need to retrieve your API key from the OpenAI dashboard. This key is essential to authenticate your requests to the DALL·E API.
2.2. Installing Required Libraries
To interact with the DALL·E API, you’ll need the official OpenAI Python library. Install it using the following command:
$ pip install openai
This will install the OpenAI package which allows your Python code to interact with the API.
2.3. Setting Up API Key
Once you’ve obtained your API key from OpenAI, you must configure it in your environment. The safest way is to store the key as an environment variable to keep it secure. Run the following command to set the environment variable (on Linux or MacOS):
$ export OPENAI_API_KEY='your-api-key-here'
Alternatively, in your Python script, you can set the API key directly like this:
import openai
openai.api_key = 'your-api-key-here'
Ensure that your key is kept private and not hard-coded in public repositories.
3. Understanding the DALL·E API
The DALL·E API allows you to perform a variety of image generation tasks through several endpoints. Here’s a breakdown of the most important features:
3.1. API Endpoints
- Image Generation: This is the main endpoint for generating images from textual descriptions. You provide a prompt (text description), and the API returns a generated image.
- Image Editing: With DALL·E 3, you can not only generate images but also edit them by providing a starting image and then applying modifications through text prompts.
- Variations: You can create multiple variations of a given image using a specific prompt, allowing you to explore different styles, compositions, and designs.
3.2. Important Parameters
- Model: Specifies which version of the model you want to use. For example, “dall-e-3” is the latest version as of now.
- Prompt: A natural language description of the image you want the model to generate.
- Size: Defines the resolution of the generated image, for example, “1024×1024.”
- n: The number of images to generate. The API can return multiple images based on a single prompt.
4. Generating Images with DALL·E
Let’s dive into how to actually generate images using DALL·E with Python.
4.1. Simple Image Generation Example
The following Python script demonstrates how to generate an image based on a text prompt.
import openai
# Set the API key
openai.api_key = 'your-api-key-here'
# Send a request to the DALL·E API
response = openai.Image.create(
model="dall-e-3",
prompt="A futuristic cityscape at sunset",
n=1,
size="1024x1024"
)
# Retrieve the image URL
image_url = response['data'][0]['url']
print(image_url)
In this example:
- model: Specifies that we are using the DALL·E 3 model.
- prompt: A detailed description of the image (“A futuristic cityscape at sunset”).
- n: Number of images to generate (we’re generating just one).
- size: The resolution of the generated image, set to 1024×1024.
The script will output a URL where you can view or download the generated image.
4.2. Saving the Image Locally
You can also modify the script to download and save the generated image to your local system.
import requests
# Get the image URL from the response
image_url = response['data'][0]['url']
# Send a GET request to fetch the image
img_data = requests.get(image_url).content
# Save the image to a file
with open("generated_image.jpg", "wb") as f:
f.write(img_data)
print("Image saved as generated_image.jpg")
4.3. Generating Multiple Images
You can modify the n
parameter to generate more than one image from a single prompt. Here’s how to generate three different images:
response = openai.Image.create(
model="dall-e-3",
prompt="A futuristic cityscape at sunset",
n=3,
size="1024x1024"
)
for i, data in enumerate(response['data']):
image_url = data['url']
img_data = requests.get(image_url).content
with open(f"generated_image_{i+1}.jpg", "wb") as f:
f.write(img_data)
print(f"Image {i+1} saved.")
This script generates three images and saves them as separate files.
5. Advanced Features and Capabilities
5.1. Image Editing with DALL·E
DALL·E 3 also supports editing existing images. By providing an initial image and a text prompt describing the desired edits, you can modify images in various creative ways.
Example use case: You can start with an image of a car and edit it by changing its color or background using a simple text prompt.
5.2. Variations
DALL·E 3 also supports creating variations of an existing image. You can use a generated image as input and request new variations that explore different artistic styles, perspectives, or compositions.
6. Best practices for effective image generation
When working with the DALL·E API, there are several best practices to keep in mind to get the most out of your experience:
6.1. Craft Clear and Specific Prompts
The more detailed and specific your prompt, the better the generated image will match your expectations. Avoid vague prompts, and try to provide as much detail as possible about what you want the model to generate.
6.2. Experiment with Image Sizes and Aspect Ratios
Adjust the size and aspect ratio to fit the needs of your application. For example, if you’re generating images for a website banner, a landscape aspect ratio may be more appropriate.
6.3. Error Handling
When integrating the DALL·E API into a larger application, it’s essential to implement error handling. Make sure to catch common exceptions such as network failures or rate limits to ensure smooth operation.
7. Integrating DALL·E into Your Applications
DALL·E can be integrated into a variety of applications, from web services and mobile apps to desktop software. You can build tools that generate custom visuals for users based on their input, offering a wide range of creative possibilities.
For web-based applications, you can build a backend that communicates with the DALL·E API, passing user inputs and displaying generated images directly on the website.
8. Troubleshooting Common Issues
If you run into issues when using the DALL·E API, here are some common problems and solutions:
8.1. Invalid API Key
Ensure that your API key is correct and that it hasn’t expired. Double-check the key in your environment variable or directly in the script.
8.2. Rate Limits
OpenAI’s API has rate limits to prevent abuse. If you exceed these limits, you’ll need to wait before making additional requests. Consider implementing retries with exponential backoff for smooth user experience.
8.3. Network Errors
Ensure that your network connection is stable. If you’re dealing with large images, downloading them may take some time, especially if your internet speed is slow.
9. Conclusion
The DALL·E API opens up exciting possibilities for AI-driven image generation and editing. By following the steps in this guide, you can start creating your own customized images from text prompts, experimenting with new features, and integrating this powerful tool into your applications. Whether you’re building a creative project, designing a website, or developing a marketing tool, the potential for innovation with DALL·E is limitless.
Start experimenting today, and unleash the full creative power of AI-driven image generation!